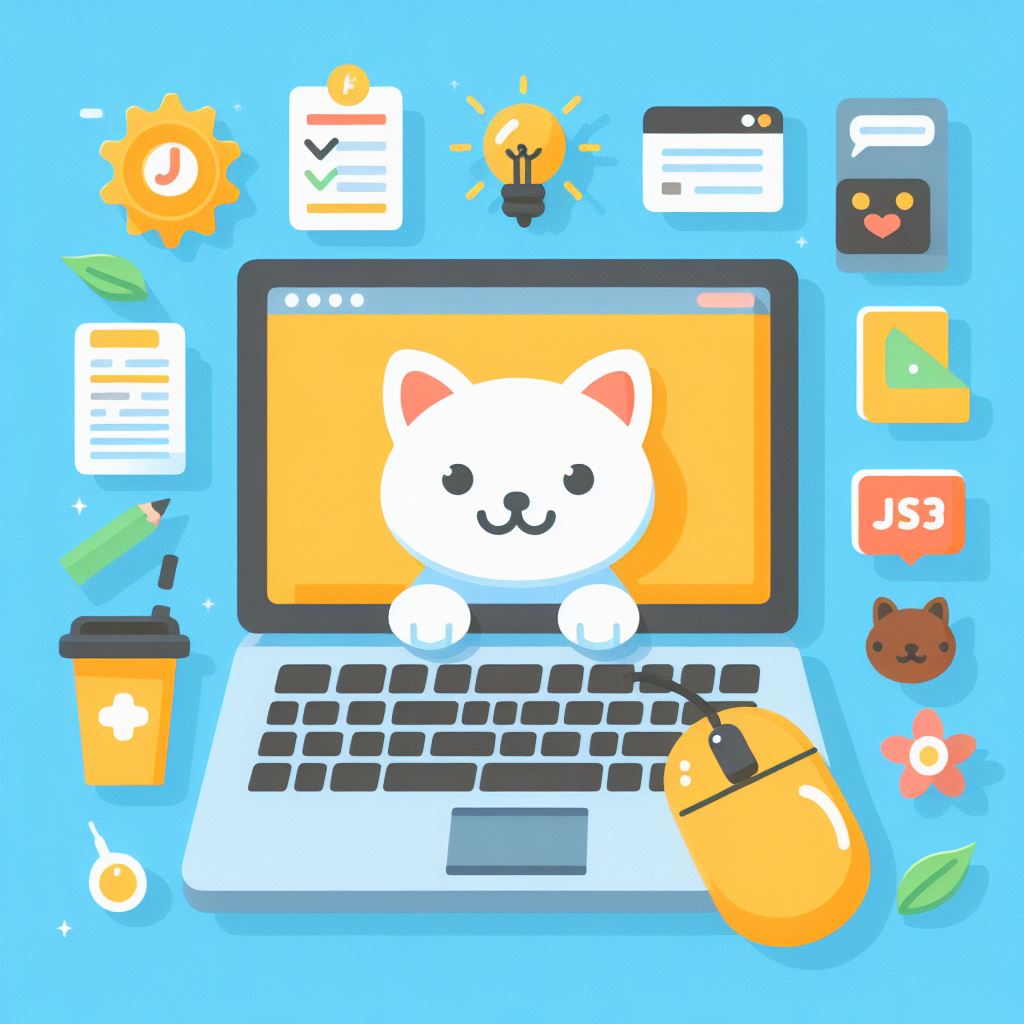
Scikit-Learn
Scikit-Learn是一個非常流行和強大的Python機器學習程式庫,提供了各種監督式和非監督式機器學習算法,以及數據預處理、模型選擇和評估等功能。以下是對Scikit-Learn的詳細介紹:
1. 安裝
Scikit-Learn可以使用Python的套件管理工具pip輕鬆安裝,只需執行:
pip install scikit-learn
2. 核心設計原則
- 一致性:所有物件都遵守相同的介面,使用和切換不同估計器變得很容易。
- 可靠性:所有估計器都是針對不同情況進行單元測試和交叉驗證。
- 可組合性:所有估計器都可以透過元估計器進行組合。
- 模型持久性:可將估計器輕鬆地保存至外部,重複使用。
3. 主要模組
- Preprocessing:特徵提取和資料預處理。
- Model Selection:設定模型超參數和選擇模型。
- Supervised Learning:廣泛的監督式學習算法,如迴歸、分類等。
- Unsupervised Learning:非監督式學習算法,如聚類和降維。
4. 範例用法
匯入資料
from sklearn import datasets
iris = datasets.load_iris()
X = iris.data
y = iris.target
訓練模型
from sklearn.linear_model import LogisticRegression
clf = LogisticRegression()
clf.fit(X, y)
預測新資料
import numpy as np
new_data = np.array([[5.1, 3.5, 1.4, 0.2]])
prediction = clf.predict(new_data)
5. 常用工作流程
- 資料載入和預處理
- 模型實例化
- 模型訓練(fit)
- 模型評估與選擇(scoring)
- 模型調參(GridSearchCV)
- 預測
6. 優點
- 易用性和一致性API
- 高效能運算
- 有效模組化設計
- 活躍的社群和文件
7. 範例
Scikit-Learn配備了許多範例,有助於快速學習。可在Python的交互式命令列環境中執行:
from sklearn import datasets, linear_model
from sklearn.model_selection import train_test_split
diabetes = datasets.load_diabetes()
data_train, data_test, target_train, target_test = train_test_split(diabetes.data, diabetes.target)
regression = linear_model.LinearRegression()
regression.fit(data_train, target_train)
總之,Scikit-Learn提供了一個簡單高效的機器學習工作流程,適合於初學者入門和專業人士運用。它涵蓋了廣泛的監督和非監督學習算法,並提供了完善的特徵處理和模型選擇功能。
以下是一些關於Scikit-Learn的重要參考資料:
官方文件:
- Scikit-Learn官方文件 (https://scikit-learn.org/stable/index.html)
- 這是最全面和最權威的Scikit-Learn參考資料,涵蓋了API說明、教學、示例、常見問題等內容。
書籍:
- 《Python Machine Learning》by Sebastian Raschka
- 《Introduction to Machine Learning with Python》by Andreas C. Müller and Sarah Guido
- 《Hands-On Machine Learning with Scikit-Learn and TensorFlow》by Aurélien Géron
線上課程:
- Machine Learning with Python (Coursera, University of Michigan)
- Python for Data Science and Machine Learning Bootcamp (Udemy)
- Machine Learning A-Z (Udemy)
教學資源:
- Scikit-Learn Tutorial (https://scikit-learn.org/stable/tutorial/index.html)
- 官方教學,涵蓋廣泛的主題,適合初學者和進階使用者。
- Scikit-Learn Video Tutorials (https://www.youtube.com/watch?v=0D5cmfRYXRU&list=PLQVvvaa0QuDfKTOs3Keq_kaG2P55YRn5v)
- Machine Learning Mastery (https://machinelearningmastery.com/start-here/#python)
社群資源:
- Stack Overflow Scikit-Learn tag
- Scikit-Learn Mailing List
- Scikit-Learn GitHub Repo
除了官方文件外,Sebastian Raschka和Aurelien Geron的書籍被廣泛推薦,提供了深入淺出的Scikit-Learn教學。Coursera、Udemy等在線課程也是不錯的學習途徑。此外,利用Stack Overflow、郵件列表等社群資源也能獲得豐富的幫助和最新資訊。